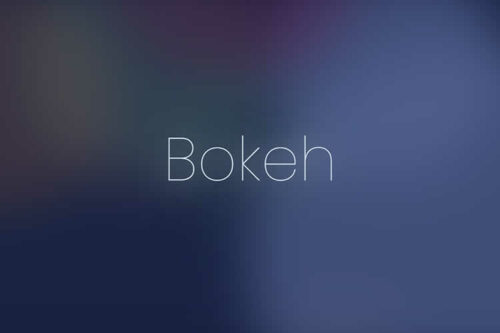

JavaScript is a relatively lightweight programming language for adding interactive functionality to web pages.
Here you will find vast collections of resources, including libraries, frameworks, plugins, snippets, and web-based tools, covering almost all areas of JavaScript development.
You might also like to browse our extensive CSS colelctions.